Published
- 5 min read
JavaScript Data Structures: Exploring Map, Set, and Object
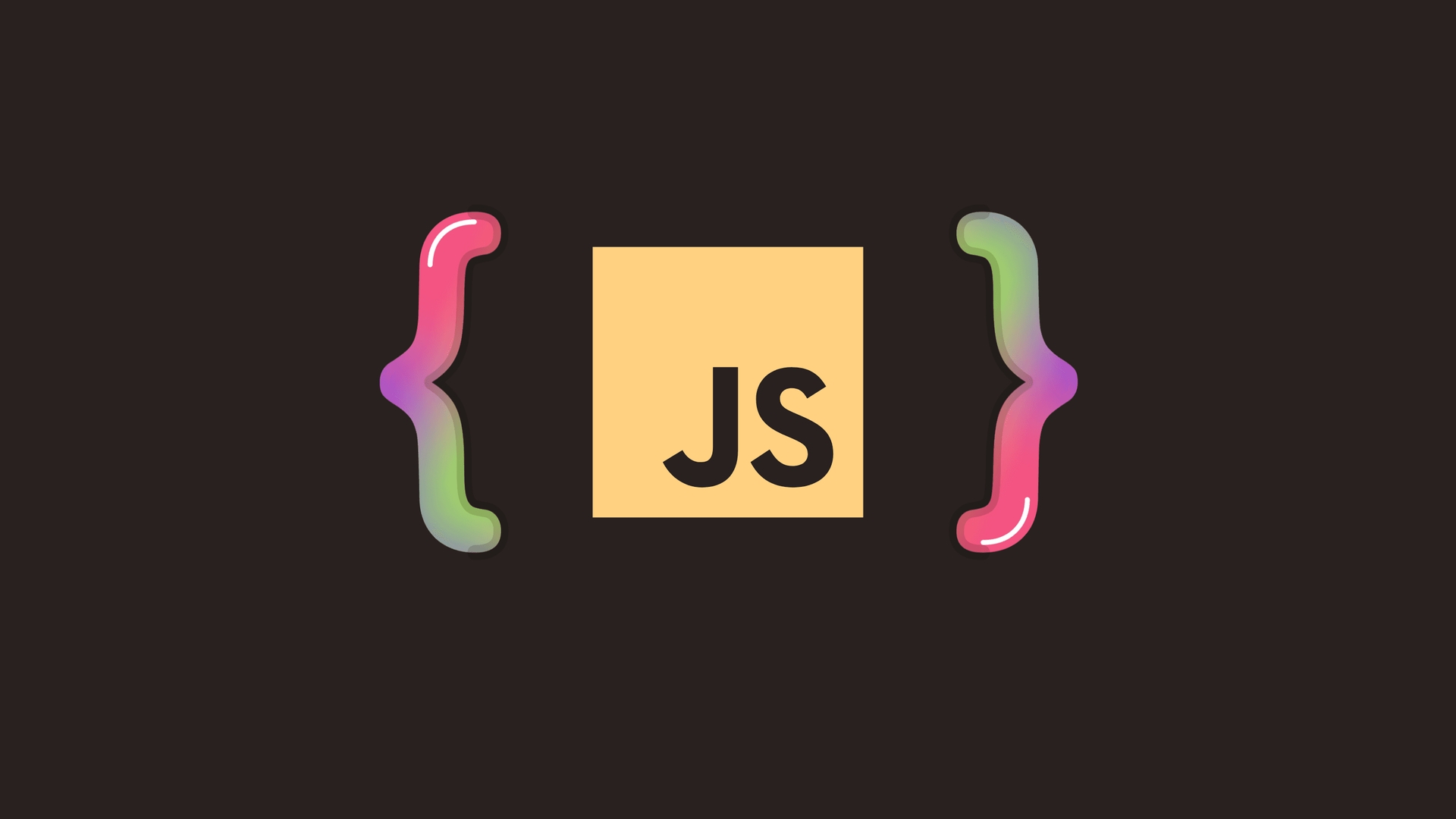
Introduction
In the realm of JavaScript, selecting the right data structure is crucial for performance optimization and readability of your code. Three of the most commonly used data structures are Map, Set, and Object. Each comes with its own distinct characteristics, uses, and trade-offs. This article delves into these three JavaScript data structures, offering a comprehensive understanding of their differences and appropriate contexts for use.
Plain Objects in JavaScript
JavaScript objects, often referred to as plain objects, are fundamental in JavaScript programming. They are key-value pairs where keys can only be strings, numbers, or symbols.
Key Characteristics of Plain Objects
- Keys are limited to strings, numbers, or symbols.
- Provide quick O(1) property access.
- Ideal for structured data and when keys are known ahead of time.
- Not required to maintain insertion order, although modern JavaScript engines commonly do.
let obj = { 1: 'one', 2: 'two', 3: 'three' }
console.log(obj[2]) // Output: "two"
JavaScript Map
A Map in JavaScript is a collection of key-value pairs, similar to an Object. However, unlike Objects, Maps accept any data type as a key.
Key Characteristics of Map
- Any data type can be a key, including objects and functions.
- Maintains the order of insertion.
- Provides better performance for frequent additions and removals.
- Includes built-in methods such as size, delete, has, get, and clear.
let map = new Map()
map.set('key1', 'value1')
map.set('key2', 'value2')
console.log(map.get('key1')) // Output: "value1"
JavaScript Set
A Set in JavaScript is a collection of unique values. It differs from Map and Object as it doesn’t work with key-value pairs, but only stores unique values.
Key Characteristics of Set
- Stores unique values of any type.
- Preserves the order of insertion.
- Contains built-in methods like add, delete, has, size, and clear.
// Define an object and a function to be used as keys
let objKey = { name: 'Object Key' }
let funcKey = function () {
console.log('Function Key')
}
// Initialize a new Map
let myMap = new Map()
// Set key-value pairs using the object and function as keys
myMap.set(objKey, 'This is the value for the object key')
myMap.set(funcKey, 'This is the value for the function key')
// Retrieve values using the object and function keys
console.log(myMap.get(objKey)) // logs "This is the value for the object key"
console.log(myMap.get(funcKey)) // logs "This is the value for the function key"
// You can also use the function key to call its function
if (myMap.has(funcKey)) {
funcKey() // logs "Function Key"
}
Deciding Between Object, Map, and Set
Determining the fastest data structure for retrieval largely depends on the context.
- Set is ideal when dealing with unique values and checking for value existence, due to its O(1) lookups.
- Map is suitable for key-value pairs where keys are not necessarily strings or symbols. Like Set, it also has O(1) lookups.
- Plain Object is a simple and fast solution when dealing with key-value pairs where keys are strings or symbols. The keys should ideally be known in advance.
The Role of WeakMap and WeakSet
JavaScript also offers WeakMap and WeakSet, similar to Map and Set, but they do not prevent their keys (WeakMap) or values (WeakSet) from being garbage-collected.
Advantages of WeakMap and WeakSet
- Prevent memory leaks in certain situations.
- Their keys/values are not enumerable, providing data privacy.
- They enable object extension without interfering with garbage collection.
FAQs
What are the key differences between JavaScript Map and Set?
JavaScript Map and Set are both iterable objects that store data in the form of [key, value] pairs. However, there are notable differences between the two. A Map is a collection of key-value pairs where the key can be any type1. On the other hand, a Set is a collection of unique values and does not operate on the key-value store concept. It’s essentially a one-dimensional array that only stores unique values1.
How are Maps different from Objects in JavaScript?
Both Maps and Objects in JavaScript are collections of key-value pairs, but there are key differences. Maps are built-in iterables, allowing the use of the for of loop or the forEach() method, which plain JavaScript Objects cannot use. Maps also have a size() method that returns the number of keys in the Map, which is not available for Objects1.
Can you give examples of when to use Set and Map in JavaScript?
Sure, let’s take a couple of examples.
For a Set, imagine there’s a meeting with people coming from different organizations. Some people come from the same organization. We need to compose a list of all the different organizations. For this, we can use a set since we only want to include every organization once1.
const organization = new Set()
organization.add('org1')
organization.add('org2')
organization.add('org3')
organization.add('org1')
organization.add('org3')
organization.add('org1')
for (let org of organization) {
console.log(org)
}
For a Map, suppose we have a pack of dogs and want to assign an age to each dog. We want to map the unique name of each dog to the age of dog1.
const dogs = new Map([
['fluffy', 10],
['barkie', 13]
])
dogs.forEach((value, key) => console.log(key, value))
How is Set different from a regular array in JavaScript?
A Set in JavaScript is a unique list of values. It’s similar to an array but can only store unique values. This means that, unlike an array, a Set cannot have duplicate values1.
var set = new Set(['a', 'a', 'e', 'b', 'c', 'b', 'b', 'b', 'd'])
console.log(set) //logs Set {"a", "e", "b", "c", "d"}
Conclusion
Understanding the differences between JavaScript’s Map, Set, and Object data structures is vital for writing efficient and readable code. Each has its own distinct characteristics and uses, and the choice between them depends on the specific use case. In general, Sets are best for unique value collections, Maps are suitable for key-value pairs with various key types, and Objects are optimal for key-value pairs with string or symbol keys. However, remember to always consider the specific needs of your code when choosing a data structure.
Hopefully, this article has provided a deeper understanding of these JavaScript data structures. Whether it’s maintaining a unique list of values with Set, mapping keys to values with Map, or using the versatility of a plain Object, each has its own strengths that can be leveraged to improve your JavaScript programming skills.